5 Best Ways to Write Concise Arrow Functions in JavaScript
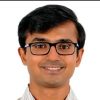
Author : Abishek Surya RS 20th May 2020
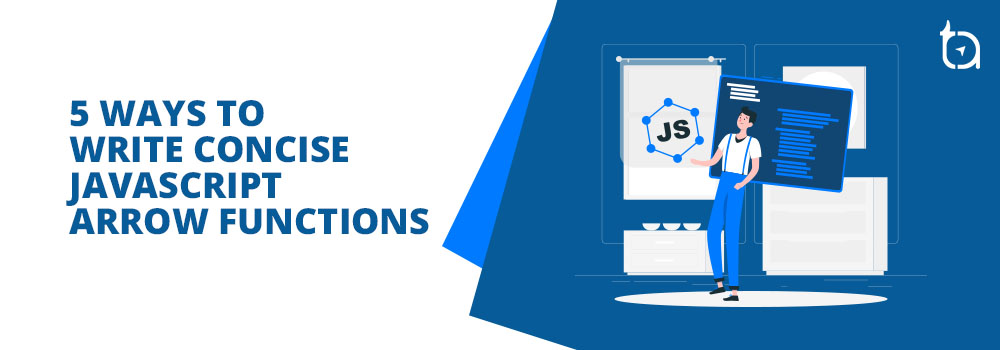
Arrow functions are very popular in JavaScriptJavaScriptJavaScript, often abbreviated as JS, is a high-level, interpreted scripting language that conforms to the ECMAScript specification. JavaScript has curly-bracket syntax, dynamic typing, prototype-based object-orientation, and first-class functions. and allow you to write shorter function syntax. Introduced in ES6, it saves a lot of development time. For example,
Normal Function
hey = func() {
return “Hey there!”;
}
After
hey = () => {
return “Hey there!”;
}
Another Way
Hey = () => “Hey there!”;
There are certain benefits that come with using arrow functions in your code.
- Arrow functions bind this to the surrounding code’s context.
- The syntax works well when there is no body-block and allows an implicit return.
- Simpler and shorter code in some cases.
- It fits great as a callback function.
Using arrow functions initially might be a little problematic due to syntax issues but once you are familiar with it, it can be really beneficial as a developer. You can use the following strategies to write great arrow functions in JavaScript.
1. Function Name Inference
The arrow function in JavaScript is anonymous. The name property of the function is an empty string.
For example,
(num=>num+1).name; // => ' '
The anonymous function is marked anonymous during a debug session. This gives you no idea about what code is being executed.
A debug session with anonymous code looks like as shown below,

The code stack trace on the right side consists of two functions that are anonymous. There is no useful information from this call stack information.
This is why function name inference is useful. The idea behind name inference in JavaScript is that you can determine the arrow function from its syntactic position. For example,
const increment=num=>num+1;
increment.name; // ‘increment’
Since the variable increment holds the arrow function, JavaScript now decides that ‘increment’ could be a good fit for that function. Thus the arrow function receives the name ‘increment’.
Check out a session that uses a function name inference
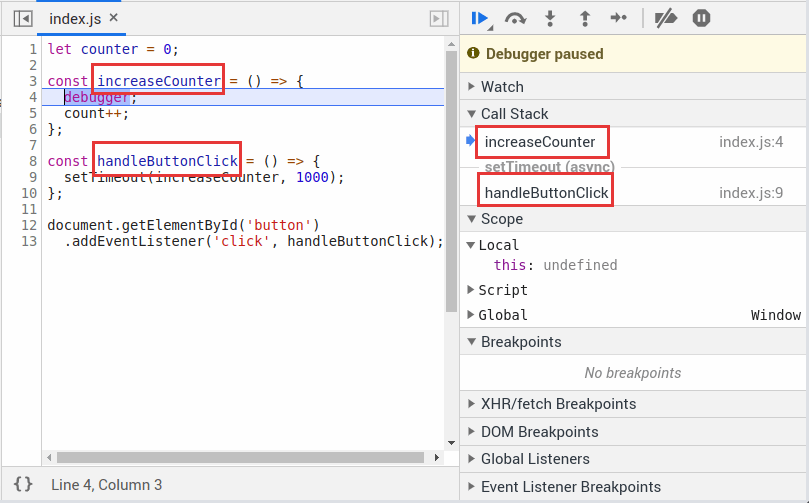
Now, since the arrow function has names, the call stack provides more information about the code being executed:
- handleButtonClick function name indicates that a button click has occurred
- incrementCounter increases the counter variable
2. Inline When it is Possible
An inline function is a function with only one expression, and they are short and save a lot of coding.
Here is an example,
const numArray=[5,10,15];
numArray.map((num)=>{
return numArray*5;
});
With the inline function, you can now remove the curly braces and return statement and make it like a single expression.
const numArray=[5,10,15];
numArray.map(num=>num*5);
3. Fat Arrow & Comparison Operators
The comparison operators like <,>,<= and >= all look similar to fat arrow => operator. So, when these operators are used in an inline function, it creates confusion. The presence of => and >= can be confusing in the same inline function.
For example,
const positiveToZero=num=>num>=0?0:num;
The first option is to wrap the comparison operator inside parentheses.
const positiveToZero=num=>(num>=0?0:num);
The second option is to define the arrow function using a longer form.
const positiveToZero=num=>{
return num>=0?0:num;
}
By using such refactorings, you can eliminate the confusion between the fat arrow and the comparison operators.
4. Declaring Plain Objects
An object literal, when declared inside an inline function, triggers a syntax error.
For example,
const numArray=[5,10,15];
numArray.map(num=>{‘num’:num});
//throws Syntax Error
The curly braces are only considered as a code block rather than an object block. Now wrapping the object literal within a pair of parentheses gives you the solution.
const numArray=[5,10,15];
//Works
numArray.map(num=>({‘num’:num}));
If the ‘object literal’ has lots of properties, you can use newlines while keeping the function inline.
const numArray=[5,10,15];
//Works
numArray.map(num=>
({‘num’:num
‘propA’:‘value A’
‘propB’:’value B’
}));
5. Avoid Excessive Nesting
Though arrow functions are short, using them repeatedly could lead to unreadable code. The code now becomes cryptic and complicated.
For example, a button is clicked here, and a request to the server is sent. When a response is ready, it is logged to the console.
myButton.addEventListener('click',()=>{
fetch('/items.json')
.then(response=>response.json())
.then(json=>{
json.forEach(item=>{
console.log(item.name);
});
});
});
There are 3 levels of nesting happening here, and it is nearly impossible to understand what is happening.
To increase readability, you need to introduce variables that hold an arrow function. The variable needs to describe exactly what the function does.
const readItemsJson=json=>{
json.forEach(item=>console.log(item.name));
};
const handleButtonClick=()=>{
fetch('/items.json')
.then(response=>response.json())
.then(readItemsJson);
};
myButton.addEventListener('click',handleButtonClick);
Now, the refactoring extracts the arrow functions into the variables readItemsJson and handleButtonClick. Now, it’s easier to understand what happens since nesting comes down from 3 to 2.
The other way is to use async/await syntax so that you can refactor the entire code.
const handleButtonClick=async()=>{
const response=await fetch('/items.json');
const json =await response.json();
json.forEach(item=> console.log(item.name));
};
myButton.addEventListener('click',handleButtonClick);
Final Thoughts
In summary, when writing arrow functions, it is important to remember these 5 best practices so that there is readability as well as efficiency in code. As a summary, here is a round-up of all that has been discussed.
- The arrow functions in JavaScript are anonymous. Use variables to hold these arrow functions to make debugging easier and productive. This aids JavaScript in inferring function names.
- An inline function is useful when the function body has only one expression.
- The comparison operators < , > , <= , >= can be easily confused with the fat arrow => operator. Use parentheses to separate them clearly when using in an inline function.
- The object literal syntax { prop: ‘ value’ } is similar to the block of code { }. When placing it inside an inline function, wrap it into a pair of parentheses. ( ) => ( { prop: ‘value’ } ).
- Finally, excessive nesting can get in the way of writing good arrow functions. To improve readability, reduce the arrow functions by extracting them into variables. Another way to do this is to use async/await features.
At TechAffinity, our JavaScript developers are some of the highly skilled and experienced professionals who can serve your business demands. In case of further queries, feel free to mail us at media@techaffinity.com or you can schedule a meeting.
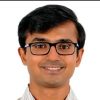