10 Important Concepts in JavaScript Functional Programming for Enhanced Product Development | Part 2
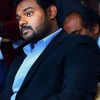
Author : John Prabhu 1st Sep 2020
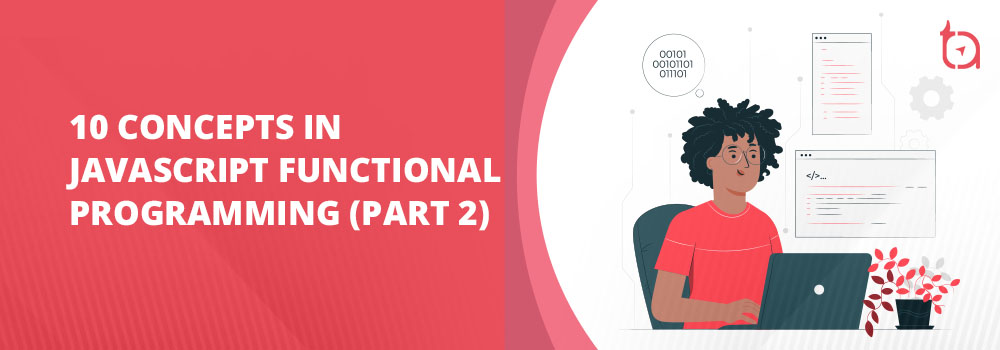
As discussed in our previous part: Functional Programming in JavaScript – Part 1, startups can’t afford to waste time on trivial errors that might happen during JavaScript development. So, here we are to continue the rest of the concepts of JavaScript Functional Programming.
1. Currying
Currying is a technique of translating a function evaluation that takes multiple parameters into evaluating multiple functions that each take a single parameter.
Let’s understand with an example:
const addition = (x,y) => x+y; | |
addition(8,9); // 17 | |
// the above function takes multiple arguments | |
const currying = (x)=>(y)=>x*y; | |
currying(6)(9); // 54 |
In the above example, we have created two functions: addition and currying. The addition function is capable of taking two parameters at a time; whereas, the currying function takes only one parameter at a time. Thus, we explored how you can convert a function with multiple parameters into multiple functions that accept one parameter at a time.
2. Partial Application
Partial Application is a method of applying a function. Suppose a function has 7 arguments. We want its execution to be partial i.e., for now we will pass 3 arguments and the rest of the 4 arguments will be passed later on. This is called partial application and it is possible due to closure. When we apply a function partially, the arguments we passed are remembered, and are used when we fully execute the function with the remaining number of arguments. Here’s an example to understand this.
const multiply = (x,y,z) =>x*y*z; | |
const partialMultip = multiply.bind(null,6); | |
partialMultip (5,2) //60 |
In the above example, partialMultip partially applies the multiply function with 6 as its first argument. When executing the partialMultip function, we only have to pass the remaining parameters as the first argument 6 has been remembered due to closure.
3. Memoization
It is a special form of caching that helps in increasing a function’s performance. Memoization caches the return values of functions corresponding to its parameter. Unless the parameter is constant, the return value is memorized. Here’s an example to understand this.
const notMemFn = (x) => { | |
return x*4; | |
} | |
notMemFn(2); // 8 | |
notMemFn(2); // 8 | |
// every time we run the function, the multiplication code get executed, which can be optimized by memoization |
const memFn = () => { | |
const cache = {}; | |
return (x) => { | |
if( x in cache ) { | |
return cache[x]; | |
} else { | |
return x*4; | |
} | |
} | |
} | |
const multiplication = memFn(); | |
multiplication(2); // 8 | |
multiplication(2); // 8 - returns from cache. As the parameter is same so the return value is memoized |
In the above example, we have used two functions notMemFn and memFn. The notMemFn function will execute the given function’s logic of multiplication for each execution even for the same parameter. On the other hand, for memFn, the function logic of multiplication will only be executed if the result is not cached. When a parameter is repeated for the second time with the same value, the output comes from cache.
4. Arity
Arity means the number of arguments the function can take in. It is preferred to have less number of arguments to a function to make it more usable. My preference for the number of arguments a function should have is 1 or 2. Let’s understand this with an example.
const addingTwoNum = (num1,num2) => num1+num2 | |
const mathAbs = (num) => Math.abs(num) | |
// addingTwoNum has arity of two | |
// mathAbs has arity of one |
In the above example, we have created two functions addingTwoNum and mathAbs. The addingTwoNum function has arity of 2 as it has two arguments and the mathAbs has arity of 1 as it has only one argument.
5. Compose and Pipe
In simple terms, compose is a concept that keeps on iterating a data through a function unless the desired output is obtained. To elaborate, when a data is processed by a function, it returns a new data. The new data will again be processed but by another function. The sequence of execution continues till we get the required output.
Though pipe is similar to compose, the difference is in execution. Compose executes the components from right to left; whereas, pipe executes the components from left to right.
Here’s an example to understand this.
// Our task is to multiply a number with 3 and take absolute of that number | |
const multiplyWith5 = (x)=>x*5; | |
const getAbsouleOfNum = (x)=>Math.abs(x) | |
// here we have two component multiplyWith5 and getAbsouleOfNum | |
// if we want to do that without compose we will do like these | |
let x = 7; | |
let xAfterMultiply = multiplyWith5(x); | |
let xAfterAbsoulte = getAbsouleOfNum(xAfterMultiply); |
//Let's do it in composable way | |
const compose = (f,g) => data=>f(g(data)); | |
const multiplyBy5andGetAbsolute = compose(multiplyWith5,getAbsouleOfNum); | |
multiplyBy5andGetAbsolute(-7) //35 |
//Let's do it in Pipe fashion | |
const pipe = (f,g) => data=>g(f(data)); | |
const multiplyBy5andGetAbsolutePipe = pipe(multiplyWith5,getAbsouleOfNum); | |
multiplyBy5andGetAbsolutePipe(-7) //35 |
In the above example, we explained how we can use compose to transform the data. The program began with a requirement to multiply a number with 5 and then get the absolute value of the number. As you can see, these are two different operations. So, we have created two functions multiplyWith5 and getAbsouleOfNum. Both of these functions are pure functions. Now, if we do not use compose, firstly, we have to call multiplyWith5 function initially and store the output in that variable. Secondly, we have to use that variable to call getAbsouleOfNum function and get the desired result. This is one way of doing it.
Let’s now try the same in the compose way. For that, we need two functions: multiplyWith5 and getAbsoluteOfNum. When you arrange these functions in a sequential fashion, the output of one function becomes the input of another. So, we have created another function “multiplyBy5andGetAbsolute” to execute the getAbsoluteOfNum and the executed output will be fed as input for the function “multiplyWith5”.
You can also do this in the pipe way. All you have to do is to create the function multiplyBy5andGetAbsolutePipe, execute the multiplyWith5 function and feed the resulting output to getAbsoluteOfNum.
We, at TechAffinity, have developers who are familiar with both OOP and functional programming paradigms. Depending on the project requirement and the deadline, we strategically leverage the skill sets from them. Our experts are capable of handling the development of your product. Feel free to share your queries with us by sending an email to media@techaffinity.com or schedule a meeting with our experts.
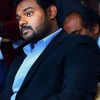