Simplify Concatenation with JavaScript String Interpolation
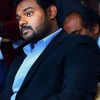
Author : John Prabhu 16th Apr 2020
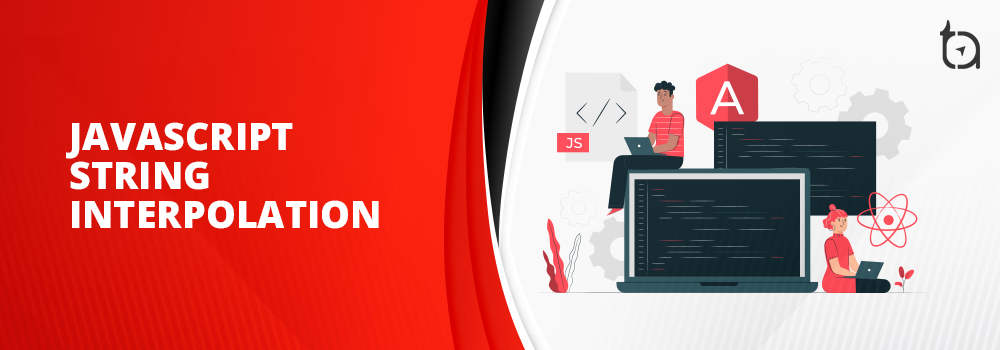
What is String Interpolation?
String Interpolation is a process that helps you substitute values of constants and variables into placeholders in a string.
There are a lot of syntaxes used for different programming languages to perform the same task. But, we use $[expression} and template literals as placeholders to perform the JavaScript string interpolation.
An Example with Constant:
const integer = 27;
const out = `The number is ${integer}`;
out;
Output:
“The number is 27”
An Example with Variable:
var orange = 4;
var watermelon = 3;
console.log(`I have ${orange} Oranges`);
console.log(`I have ${watermelon} Watermelons`);
console.log(`So, totally I have ${orange + watermelon} fruits`);
Output:
I have 4 Oranges
I have 3 Watermelons
So, totally I have 7 fruits
Here, we are going to look into some examples using constants and best practices of how to use template strings to perform JavaScript string interpolation.
1. The String Literals
You can create string literals using three different ways:
1. You can wrap the string into a pair of single quotes ‘
const text = ‘TechAffinity – The Best IT Services Company in Tampa’;
2. You can wrap the string into a pair of double quotes “
const text = “TechAffinity – The Best IT Services Company in Tampa”;
3. You can wrap the string into a pair of backticks `
const text = `TechAffinity – The Best IT Services Company in Tampa`;
Among these 3, the string literal wrapped in backticks is also called a template string and it supports string interpolation.
2. The placeholders
The template string supports placeholders and the expressions inside the placeholders are processed when the program runs. As a result, the string is inserted into the placeholder.
The format of a placeholder looks like this: ${expressionToProcess} and this expression can be of any kind.
variables: ${Var}
operators: ${a1 + a2}, ${cond ? ‘val 1’ : ‘val 2’}
function calls ${myFunc(‘argument’)}
Here’s an example:
const company = 'TechAffinity';
const city = 'The Best IT Services Company in Tampa';
const out = `${company} - ${city}`;
out;
Output:
“TechAffinity – The Best IT Services Company in Tampa”
`${company}, ${city}!` (the backticks) is a template string having placeholders ${company} and ${city}.
During the execution of the script, the first placeholder ${company} is replaced with the value of Company variable, and the same for ${city}. The string interpolation result is ‘TechAffinity – The Best IT Services Company in Tampa’.
You can write in a number of expressions inside a placeholder such as a function call, an operator, and also complex expressions.
const a1 = 5;
const a2 = 10;
const value = `The sum is ${a1 + a2}`;
value;
Output:
‘The sum is 15’
const a1 = 5;
const a2 = 10;
function mult(no1, no2) {
return no1 * no2;
}
const out = `The total is ${mult(a1, a2)}`;
out;
Output:
‘The total is 50
$(a1 + a2) is a placeholder consisting of the multiplication operators and 2 operands. ${mult(n1, n2}) contains a function invocation.
2.1 Implicit to String Conversion
The placeholder expression output is converted into a string. For instance, a number in a placeholder will be transformed into a string in the below example:
const x = 11.2;
const out = `The number is ${x}`;
out;
Output:
`The number is 11.2`
The expression x of the placeholder ${x} is taken as number 11.2. The number 11.2 is then transformed into a string ‘11.2’, and included in the interpolation result: ‘The number is 11.2’.
When a conversion to string rule is in place and a placeholder contains an object, the object gets converted to a string. By calling the toString() method, you can get the string representation of the object.
Below is an example of inserting an arrays into a template string:
const array = [3, 5, 7, 11];
const out = `The numbers are ${array}`;
out;
Output:
“The numbers are 3,5,7,11”
The placeholder ${array} contains an array of odd numbers.
toString() array method also carries out array.join(‘,’) when the array is converted to string. Hence, the string interpolation result is “The numbers are 3,5,7,11”
3. Escaping placeholders
Because the placeholder format ${expression} has a special meaning in the template literals, you cannot use the sequence of characters “${someCharacters}” without escaping.
By now, you will be familiar with the placeholder format ${expression} and its functionality in template literals. Hence, you can’t use a normal text that resembles the same format without processing it as a template literal.
For instance, here’s an example that has the sequence of characters ${xyz}.
const out = `a random expression ${xyz}`;
out;
Output:
“ReferenceError: xyz is not defined”
Coding in ${xyz} results in an error because JavaScript interprets ${xyz} as a placeholder.
Hence, you can use a backslash “ \ ” before the sequence that resembles the placeholder format and it solves the problem:
const out = `a random expression \${xyz}`;
out;
Output:
“a random expression ${xyz}”
In the template string `a random expression \${xyz}` JavaScript doesn’t interpret \${xyz} as a placeholder now, but as a character sequence.
Alongside with ${xyz}, the sequence of characters like ${xyz and ${ also have to be escaped with a backslash \:
Here’s another interesting example where the backslash can save even the incomplete expressions as discussed below:
const out = `a random expression \${xyz} \${xyz \${`;
out;
Output:
‘A random expression ${xyz} ${xyz ${‘
4. Best practices
4.1 Refactor String Concatenation
When you want to concatenate longer strings, you must use string interpolation and not string concatenation.
Let’s see how complex it is to concatenate string literals and how easy it is to use template strings with below two illustrations:
Illustration 1:
const a1 = 10;
const a2 = 30;
const out = 'The multiplication of ' + a1 + ' and ' + a2 + ' is ' + (a1 * a2);
out;
Output:
“The multiplication of 10 and 30 is 300”
Then it’s the time to switch to JavaScript string interpolation using template strings:
Illustration 2:
const a1 = 10;
const a2 = 30;
const out = `The multiplication of ${a1} and ${a2} is ${a1 * a2}`;
out;
Output:
“The multiplication of 10 and 30 is 300”
Thus, you can perform the same operations using template strings with less code that is easy to comprehend as well.
4.2 Helper Variables
If your code happens to include more template strings with more complex expressions, it might negatively impact the readability of the literal.
Below is an example of placeholders with complex expressions:
const a1 = 7;
const a2 = 3;
const message = `Add: ${a1 + a2}, Sub: ${a1 - a2}, Power: ${Math.pow(a1, a2)}`;
message;
Output:
“Add: 10, Sub: 4, Power: 343”
The more complex the placeholders are, the more tempting is to add helper variables to store intermediate values.
To improve readability, we can include helper variables to store the intermediate values.
const a1 = 7;
const a2 = 3;
const add = a1 + a2;
const sub = a1 - a2;
const power = Math.pow(a1, a2);
const out = `Add: ${add}, Sub: ${sub}, Power: ${power}`;
out;
Output:
“Add: 10, Sub: 4, Power: 343”
Thus, the template string becomes more readable now than before when the helper variables such as add, sub and power are put in use. Moreover, when intermediate variables are used, the code-documents it.
4.3 Single quotes in placeholders
I recommended using single quotes ‘ rather than backticks ` in the expressions inside the placeholder.
It is generally recommended to use single quotes instead of backticks in the expression inside the placeholder. It is so because too many backticks make it difficult to comprehend the code.
Here’s an example from a code snippet:
function getLoadingMessage(isLoading) {
return `Data is ${isLoading: `loading...` : `done!`}`;
}
For a more a cleaner and comprehensive look, we have used single quotes as shown below:
function getLoadingMessage(isLoading) {
return `Data is ${isLoading: 'loading...' : 'done!'}`;
}
5. String interpolation in TypeScript
The string interpolation process in TypeScript is similar to the process followed in JavaScript. The only thing you need to consider in this case is that the TypeScript will be converted into equivalent JavaScript code before processing.
TypeScript Code:
const number: num = 10;
const text: message = `You have ${number} products`;
text;
Equivalent JavaScript Code:
const number = 10;
const out = `You have ${number} products`;
out;
Output:
‘You have 10 products’
Final Thoughts
The JavaScript string interpolation is definitely a useful feature as it helps you to store values inside string literals in a comprehensive way and avoid using the complex string concatenation approach. Also, you don’t have to complicate the string literal. Make use of intermediate variables to store expressions before inserting them into placeholders and avoid the template string complexities as and when required.
We, at TechAffinity, have expert developers who can simplify a lot of complex programming processes that result in improved productivity without compromising on efficiency. Our experts develop robust codes that offer a lot of room to make future changes and be ahead of your competition’s track. If you’re on the lookout for JavaScript developers who are exceptional in solving problems, line up your queries to media@techaffinity.com or schedule a meeting with our developers.
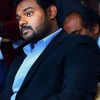