What is Lazy Loading & How to do it?
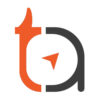
Author : TechAffinity 28th Oct 2020
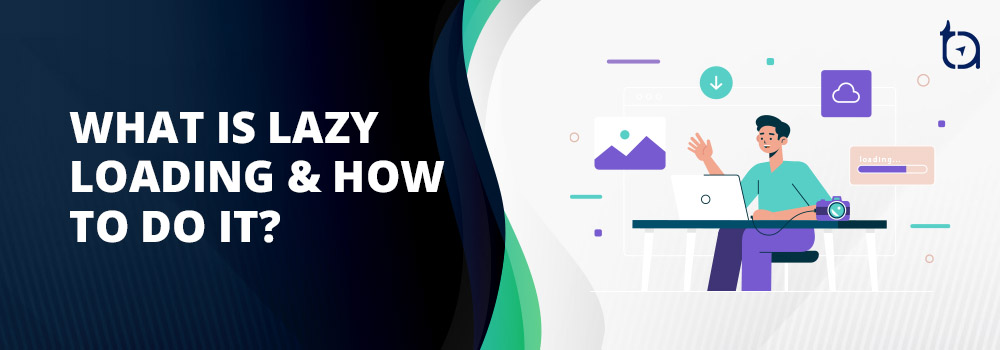
Images are a critical component of any website. Be it any kind of website, it is impossible to imagine it without images. But you need to understand that heavy images can make your website slow and your visitors might end up with a bad user experience. To ensure this problem is addressed, lazy loading was introduced. It was done to make sure that loading times of the web pages were significantly reduced.
For example, on a page that is long, images at the bottom sections might be given a little more time to load. The images above the fold need to be loaded as soon as possible. You want to retain the attention of the user and ensure there is no waiting time. This can ensure a better user experience, website performance, and faster loading times. You can also implement lazy loading in such a way that any image on your website is loaded only after the successful loading of text content.
We will be discussing lazy loading and why you must choose lazy loading, the best practices involved, and the most popular JavaScript libraries used for lazy loading.
What is Lazy Loading?
Lazy loading refers to deferring loading images to a later point in time than loading them all upfront. The images after the fold loads conditionally or only when they are on the browser’s viewport. If your users don’t scroll down, then you can skip loading them. You can lazy load images on an image-heavy website to save the number of images that need to be loaded upfront.
To check this out, you can head over to Google Images and search for something like, “Mobile Application Development.” Then scroll down to the bottom of the page and check how lazy loading works. They are loaded as they become available to the user’s viewport.
Why Should You Implement Lazy Loading?
- Better Performance
There is an improvement in the loading time of your website. With lazy loading, the amount of data that gets downloaded comes down. When this happens, you end up loading fewer images on the website, resulting in better performance of the website and better utilization of resources.
- Lower Cost
It helps website administrators in getting the cost reduced by transferring a lesser amount of data (bytes) across Content Delivery Networks. These networks charge for every byte of data, and the lesser the data transferred, the lower the cost. When the data does not reach these networks due to fewer requests, delivery costs come down. You can make use of the Google Lighthouse Audit tool to choose images ideal for lazy loading. Also, you have Imagekit’s Website Analyzer to perform website optimization.
- SEO
Is Lazy Loading Bad for SEO? We believe lazy loading is, without a doubt, necessary for your website. Even Google promotes lazy loading in its Developers Guide :
“As far as performance improvement techniques go, lazy loading is reasonably uncontroversial. If you have a lot of inline imagery in your site, it’s a perfectly fine way to cut down on unnecessary downloads. Your site’s users and project stakeholders will appreciate it!”
Good SEO means your website loads faster, and users prefer fast loading websites. It means your bounce rate will be lower, and PageSpeed rankings will improve on Google. Now, Google finds your page much more relevant. You end up getting many more visitors and improving conversions on your website.
- User Experience
A fast loading website is better than a slower one. With the high competition, it becomes essential to save your visitors as much bandwidth and time as possible. If you ensure your visitors get quick access to only what’s required, it will boost their chances of coming back to your website. You also save the system resources for your visitors, specifically battery and memory, which can make them come back to your website once again.
Lazy Loading Best Practices
- Consider the Fold
Though there is a temptation to lazy load every element of your website, it is not advisable. Do not lazy load images that are above the fold. These are critical assets of your website, and you can load them as usual. The ones above the fold should be displayed using the standard <img> element as soon as possible.
Is there a foolproof way to determine the threshold value for the fold? With devices of varying shapes and sizes, you might need to consider going with a buffer value of some distance. For example, the Intersection Observer API allows you to specify a rootMargin property in an options object when you create a new IntersectionObserver instance.
let lazyImageObserver = new IntersectionObserver(function(entries, observer) {
// lazy-loading image code goes here
}, {
rootMargin: “0px 0px 256px 0px”
});
As you can see, the bottom value of the observed element has been shifted by 256 pixels. Now, the callback function will begin to execute when the image is within 256 pixels of the viewport. For those browsers not supporting this API, use scroll event code and adjust getBoundingClientRect check to include a buffer.
- Layout Shifting and Placeholders
When you lazy load images, you run the risk of triggering changes that might be expensive on the system. Users also might be inconvenienced with the layout changes. So, it is better to come up with a more consistent interface. You can consider having a placeholder with the same dimensions as the final image. Techniques such as LQIP or SQIP will be useful for this purpose. You need to indicate the presence of a media content that is about to load.
For <img> tags, make the src attribute point to the placeholder before the attribute redirects to the final image URL. In the case of <video> tags, the poster attribute points to the placeholder image. The height and width attributes need to be used on both <img> and <video> tags to ensure smooth transitioning from a placeholder image to the final image as the media content loads to keep the rendered size of the element.
- Image Decoding Delays
At times, when you lazy load images into the DOM, there might be issues that might cause the user interface to become unresponsive for some time. The main thread might become busy decoding images, and this might lead to such situations. To offset this, you can use the decode method before inserting such images into the DOM. For example,
var lazyImage = new Image();
lazyImage.src = “my-lazy-image.jpg”;
if (“decode” in lazyImage) {
// Fancy decoding logic
newImage.decode().then(function() {
imageContainer.appendChild(lazyImage);
});
} else {
// Regular image load
imageContainer.appendChild(lazyImage);
}
- Resources Fail to Load
When images fail to load, you need to be ready with a contingency plan. For example, you have a user who has left a tab open for several hours. During this time, you might have done a new deployment of the website. It could possibly lead to a change in the image’s name. So, it might not appear when the user reloads the browser again. For such cases, you need to have back up plans to prevent image fails.
Consider this solution,
var lazyImage = new Image();
newImage.src = “my-lazy-image.jpg”;
lazyImage.onerror = function(){
// Decide what to do on error
};
newImage.onload = function(){
// Load the image
};
- JavaScript Availability
Sometimes, JavaScript might not be available. In such cases, <noscript> markup can be used. Consider the following piece of code,
<!– An image that eventually gets lazy-loaded by JavaScript –>
<img class=”lazy” src=”placeholder-image.jpg” data-src=”image-to-lazy-load.jpg” alt=”I’m an image!”>
<!– An image that is shown if JavaScript is turned off –>
<noscript>
<img src=”image-to-lazy-load.jpg” alt=”I’m an image!”>
</noscript>
If JavaScript is not available, visitors will see both the placeholder image as well as the one inside <noscript> elements. You can overcome this by adding the following code to <html> tag, <script> tag, and some CSS to hide elements with lazy class.
<html class=”no-js”>
<script>document.documentElement.classList.remove(“no-js”);</script>
.no-js .lazy {
display: none;
}
Visitors with JavaScript turned off might not stop getting placeholder images but they will stop getting something that is not meaningful content.
Popular JavaScript Libraries for Lazy Loading
Here is a quick summary of the most popular and platform-based plugins that would allow users to implement lazy loading with ease instead of developing it from scratch.
- Yet Another Lazy Loader – This library uses Intersection Observer API and falls back to event-based lazy loading for browsers that do not support it. It does not work on background images in CSS. It supports IE versions back to IE11.
- WordPress A3 Lazy Load – Out of the several WordPress plugins, it comes with a lot of features and includes a fallback when JavaScript is unavailable.
- lazysizes – A popular library with extensive functionalities and supports responsive images. It provides superior performance though it does not use Intersection Observer API.
- jQuery Lazy Load – A library with jQuery implementation.
- WeltPixel Lazy Loading Enhanced – A Magento 2 extension.
- Shopify Lazy Image Plugin – Enable lazy loading on a Shopify site.
Final Thoughts
Lazy loading is still highly relevant and it is up to you to use it for your website optimization. It provides a way to increase loading speeds, improve your website performance, and cut down on your overall costs.
We, at TechAffinity, have experts who have in-depth knowledge of image optimization techniques and website development methods currently being used. In case of further queries, shoot an email to media@techaffinity.com or schedule a call with our expert team.