Object-Oriented Programming in JavaScript Explained | Should a Startup go with OOP?
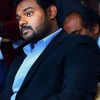
Author : John Prabhu 7th Jul 2020
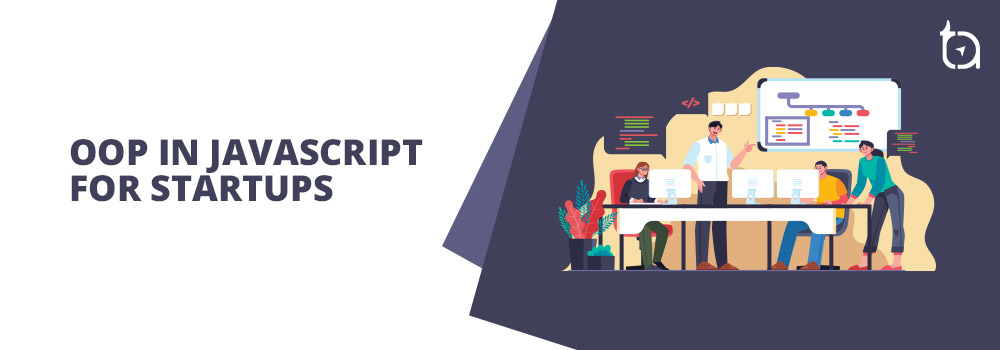
As a CTO of a software-based startup, you’ll be starting from scratch while building a new software product for your startup. For the new product, should you be choosing functional programming languages such as Haskell, Scala, etc. or Object-Oriented Programming (OOP) languages such as JavaJavaJava is one of the majorly used general-purpose programming language designed to have no or limited implementation dependencies. Java requires a software platform for its compiled programs to be executed. Oracle and Android SDK are a few examples of the software platforms on which Java executes its programs., PythonPythonWith dynamic semantics, Python is an object-oriented, interpreted, high-level programming language. Python reduces the maintenance cost of the program as it has simple and easy to learn syntax that emphasizes readability. Thanks to its high-level built-in data structures for making it attractive for Rapid Application Development, as well as for using it as a scripting language. Python supports a wide range of libraries and a few of them are numpy, pandas, matpotlib, scipy, etc., etc.? Also, there are programming languages such asJavaScriptJavaScriptJavaScript, often abbreviated as JS, is a high-level, interpreted scripting language that conforms to the ECMAScript specification. JavaScript has curly-bracket syntax, dynamic typing, prototype-based object-orientation, and first-class functions. that supports multiple paradigms. Let’s take a look at the OOP paradigm of JavaScript today.
Object-Oriented Programming in JavaScript:
An object is a front-end element with which you can enable interactivity of your website or web app and the object has properties and methods. Regardless of the programming language, the object is the heart of the OOP paradigm. So, you need to look for self-sufficient objects and not individual variables and functions.
Here’s a list of concepts that are most often used when implementing OOP:
- Object, property, and method
- Class
- Encapsulation
- Abstraction
- Reusability/inheritance
- Polymorphism
- Association
- Aggregation
- Composition
Let’s discuss them all below with JavaScript code examples.
Object, Property & Method:
Object literal
You can create a new object by defining its properties inside curly braces in JavaScript. Object literal property values can be of any data type such as arrays, strings, function literal, numbers, or Booleans. Below is an example object with a name smartphone with properties such as model, brand, and year.
Once you create an object, you can get its values using dot notation. For instance, you can get the value of the model with smartphone.model. You can also access the properties using square brackets: smartphone[‘model’]
Object Constructor
Object Constructor is similar to a regular function and it will be called whenever an object is created. You can use them in your codes with the ‘new’ keyword. Object constructor come handy when you want to create multiple objects with the same properties and methods, but different values.
If you want to create multiple book objects without using an object constructor, you need to duplicate the code for each book as illustrated above. It is a time consuming process and hence, you can use object constructor to reuse the object literal.
smartphone1 and smartphone2 created an instance of smartphone and assigned it to a variable. You can also check whether an object is an instance of another object by using ‘instanceof’.
smartphone1 instanceof smartphone
// output is: true
Object.create()
Every object in JavaScript will be created from the master object only. So, when you use the word “Object” with capital ‘O’, it will refer to your master object. Also, you can print the master object in the console. The master object has a number of methods and let’s begin with Object.create() method. The Object.create() method helps you create a new object by using an existing object as the prototype. With reference to Mozilla, here’s the syntax for your reference:
Object.create(proto, [propertiesObject])
proto: The object which should be the prototype of the newly-created object.
propertiesObject: It is optional
Let’s look at a simple example for further understanding:
In the above example, you can see the summary function is specified inside the proto object. After that, you need to create a prototype object smartphone1 and assign a value for the model and brand.
We will further look at the Object.create() method in detail below.
Class
Class is not an object but is the blueprint of an object. Classes are special functions. You can define functions using function declarations and expressions and similarly, you can define classes as well. Therefore, you can create umpteen numbers of objects using the blueprint.
You can use the ‘class’ keyword and the name of the class and the syntax is similar to that of Java. Class syntax is an incredible way to leverage object-oriented programming to the fullest and manage prototypes. Here’s an example program:
Using Class Syntax:
Class syntax is “syntactical sugar” and behind the screen, it uses prototype-based models only. In simple words, classes are functions and functions are objects in JavaScript.
Encapsulation
Encapsulation is a method to hide information or data. It enables the object to execute its functionality without revealing the execution details to the caller. Simply put, the private variable can be accessed only by the current function and it is not available for global scope or other functions.
In the above-example, the ‘model’ and the ‘brand’ are visible inside the scope of the function ‘smartphone’ and the method summary is visible to the caller of ‘smartphone’. Hence, the model and the brand are encapsulated inside ‘smartphone’.
Abstraction
Abstraction is the process of hiding the implementation. Using this concept, you can hide the implementation details and only show essentials to the caller. Simply put, it hides irrelevant information and displays only what’s necessary. If you lack abstraction in your code, it will cause code maintainability problems.
Reusability/Inheritance
Inheritance is a concept that enables you to create a new class with the help of an existing class. In simple words, the child class inherits all the behaviors and properties of the parent class.
In general, JavaScript is not a class-based language. Since the introduction of the keyword ‘class’ in ES6, it supports classes, which is “syntactical sugar”. Deep down, JavaScript is a prototype-based language. In JavaScript, inheritance is achieved by using the prototype and this pattern is referred to Behavior Delegation Pattern or prototypal inheritance.
Let’s consider our book example:
Prototypal Inheritance
For each instance of Book, we’re recreating the memory for the methods from the base class. These methods must be shared across all instances — they should not be specific to the instance. Here the prototype comes into the picture:
Polymorphism
The concept of polymorphism offers a way to perform a single action in different forms, and enables you to call the same method on different JavaScript objects.
Relationships between the objects will be defined by Association, Aggregation, and Composition.
Association
Every object in JavaScript is independent and ‘Association’ is the relationship between two or more objects. Simply put, association defines the multiplicity between objects: one-to-one, one-to-many, many-to-one, many-to-many.
smartphone1 was assigned to the property of multiplicity to object smartphone2. It shows the relationship between objects smartphone1 and smartphone2. Both can be added and removed independently.
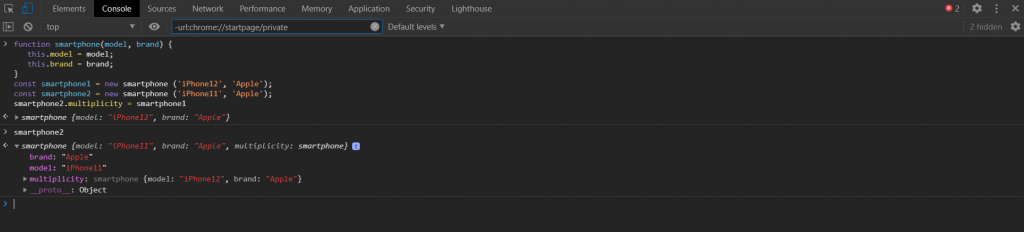
Aggregation
When an object is formed in association with a collection of sub objects, it is referred to as aggregation. In simple words, when an object contains other objects, then it is aggregation. Each sub object has its own reference identity and you can destructure them from the aggregation without any loss in information. Examples: arrays, trees, etc.
smartphone1 and smartphone2 were added to models under event object. If the event object is deleted, until smartphone1 and smartphone2 are available, then both smartphone and event live independently.

Composition
As you can see that aggregation is a special case of association, composition is a special case of aggregation. The concept of composition is: when an object contains another object and the contained object does not function properly without the container object.
Here the property event is strictly bounded with the smartphone object, and the event can’t live without the smartphone object. If the smartphone object id is deleted, then the publication will also be deleted.
Composition over Inheritance
As discussed earlier, the concept of inheritance is: when an object is based on another object. In the below example, we will look at the object ‘smartphone1’ inheriting the properties and methods from the object ‘smartphone’ such as, ‘model’, ‘brand’, and ‘summary’. As a result, it creates a relationship between ‘smartphone1’ and ‘smartphone’.
The concept composition involves collection of simple objects and combining them to form more complex objects. To create ‘book1’ we need methods. With the help of methods, you can create a relationship between ‘book1’ and ‘Book’.
Final Thoughts
You can’t take the factor time-to-market with a pinch of salt. All your decisions should certainly revolve around this factor. If you’ve a very short deadline and a couple of expert OOP developers are in your team, it is ideal to get started with them rather than waiting for experts in functional programming.
We, at TechAffinity, have developers who are familiar with the Object-Oriented Programming paradigm. Our experts are capable of handling the development of your product. Feel free to share your queries with us by sending an email to media@techaffinity.com or schedule a meeting with our experts.
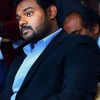