An Introduction to OOPS Concepts in Java | Abstraction Inheritance Polymorphism
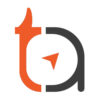
Author : TechAffinity 16th Jun 2023
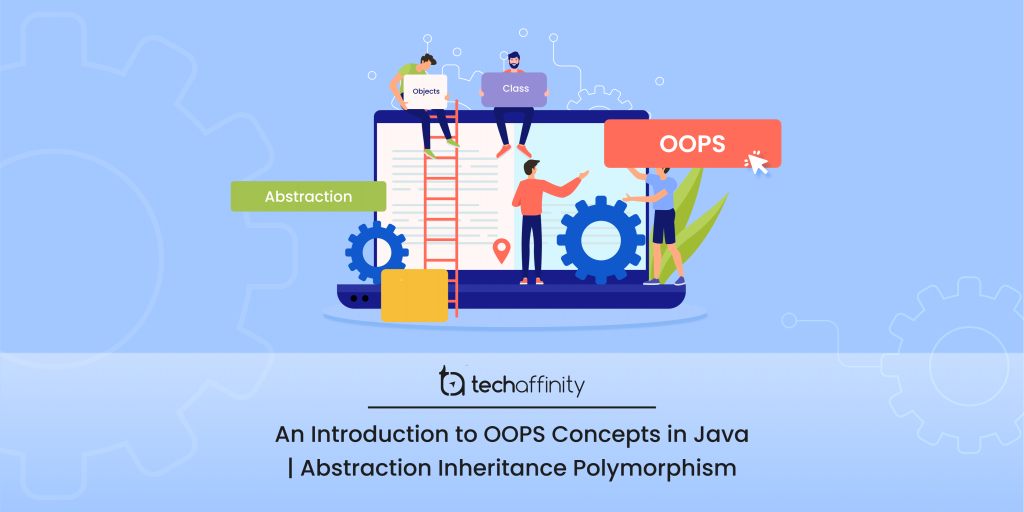
Why do we need OOPS Concepts in Java?
Object-Oriented Programming & System (OOPSOOPSObject-oriented programming is a programming paradigm based on the concept of "objects", which can contain data, in the form of fields, and code, in the form of procedures.) concepts in Java helps reduce code complexity and enables the reusability of code. Programmers feel like working with real-life entities or objects. Object-oriented programming is a programming paradigm that brings together data and methods in a single entity called object. This promotes greater understanding as well as flexibility and maintenance of code over a long period of time.
According to Statista’s “Most used programming languages among developers worldwide” survey, JAVAJavaJava is one of the majorly used general-purpose programming language designed to have no or limited implementation dependencies. Java requires a software platform for its compiled programs to be executed. Oracle and Android SDK are a few examples of the software platforms on which Java executes its programs. had a whopping 41.1% in early 2019.
JavaJavaJava is one of the majorly used general-purpose programming language designed to have no or limited implementation dependencies. Java requires a software platform for its compiled programs to be executed. Oracle and Android SDK are a few examples of the software platforms on which Java executes its programs. is a fast, secure, and reliable programming language, first released in 1995 by Sun Microsystems. Ever since it has expanded in its reach and functionality. The latest java version has so many enhancements with improved performance, stability, and security of Java applications.
What are the OOPS Concepts in Java?
1. Objects & Classes
Objects are the basic unit of OOPS representing real-life entities. They are invoked with the help of methods. These methods are declared within a class. Usually, a new keyword is used to create an object of a class in Java.
Class is a predefined or user-defined template from which objects are created. It represents properties/methods that are common to all objects of the same class. It has several features, such as access modifiers, class names, interfaces, and class bodies.
2. Abstraction
Abstraction means showing only the relevant details to the end-user and hiding the irrelevant features that serve as a distraction. For example, during an ATM operation, we only answer a series of questions to process the transaction without any knowledge about what happens in the background between the bank and the ATM.
Example Program of Abstraction in Java
abstract class Bike
{
Bike()
{
System.out.println("The Street Bob. ");
}
abstract void drive();
void weight()
{
System.out.println("Light on its feet with a hefty : 630 lbs.");
}
}
class HarleyDavidson extends Bike
{
void drive()
{
System.out.println("Old-school yet relevant.");
}
}
public class Abstraction
{
public static void main (String args[])
{
Bike obj = new HarleyDavidson();
obj.drive();
obj. weight();
}
}
Output
The Street Bob.
Old-school yet relevant.
Light on its feet with a hefty : 630 lbs.
3. Encapsulation
Encapsulation is a means of binding data variables and methods together in a class. Only objects of the class can then be allowed to access these entities. This is known as data hiding and helps in the insulation of data.
Example Program of Encapsulation in Java
class Encapsulate
{
private String Name;
private int Height;
private int Weight;
public int getHeight()
{
return Height;
}
public String getName()
{
return Name;
}
public int getWeight()
{
return Weight;
}
public void setWeight( int newWeight)
{
Weight = newWeight;
}
public void setName(String newName)
{
Name = newName;
}
public void setHeight( int newHeight)
{
Height = newHeight;
}
}
public class TestEncapsulation
{
public static void main (String[] args)
{
Encapsulate obj = new Encapsulate();
obj.setName("Abi");
obj.setWeight(70);
obj.setHeight(178);
System.out.println("My name: " + obj.getName());
System.out.println("My height: " + obj.getWeight());
System.out.println("My weight " + obj.getHeight());
}
}
Output
My name: Abi
My height: 70
My weight: 178
4. Inheritance – Single, Multilevel, Hierarchical, and Multiple
Inheritance is the process by which one class inherits the functions and properties of another class. The main function of inheritance is the reusability of code. Each subclass only has to define its features. The rest of the features can be derived directly from the parent class.
Single Inheritance – Refers to a parent-child relationship where a child class extends the parent class features. Class Y extends Class X.
Multilevel Inheritance – Refers to a parent-child relationship where a child class extends another child’s class. Class Y extends Class X. Class Z extends Class Y.
Hierarchical Inheritance – This refers to a parent-child relationship where several child classes extend one class. Class Y extends Class X, and Class Z extends Class X.
Multiple Inheritance – Refers to a parent-child relationship where one child class is extending from two or more parent classes. JAVAJavaJava is one of the majorly used general-purpose programming language designed to have no or limited implementation dependencies. Java requires a software platform for its compiled programs to be executed. Oracle and Android SDK are a few examples of the software platforms on which Java executes its programs. does not support this inheritance.
Example Program of Inheritance in Java
class Animal
{
void habit()
{
System.out.println("I am nocturnal!! ");
}
}
class Mammal extends Animal
{
void nature()
{
System.out.println("I hang upside down!! ");
}
}
class Bat extends Mammal
{
void hobby()
{
System.out.println("I fly !! ");
}
}
public class Inheritance
{
public static void main(String args[])
{
Bat b = new Bat();
b.habit();
b.nature();
b.hobby();
}
}
Output
I am nocturnal!!
I hang upside down!!
I fly !!
5. Polymorphism – Static and Dynamic
It is an object-oriented approach that allows the developer to assign and perform several actions using a single function. For example, “+” can be used for addition as well as string concatenation. Static Polymorphism is based on Method Overloading, and Dynamic Polymorphism is based on Method Overriding.
Example Program of Static Polymorphism with Method Overloading
Method Overloading
class CubeArea
{
double area(int x)
{
return 6 * x * x;
}
}
class SphereArea
{
double area(int x)
{
return 4 * 3.14 * x * x;
}
}
class CylinderArea
{
double area(int x, int y)
{
return x * y;
}
}
public class Overloading
{
public static void main(String []args)
{
CubeArea ca = new CubeArea();
SphereArea sa = new SphereArea();
CylinderArea cia = new CylinderArea();
System.out.println("Surface area of cube = "+ ca.area(1));
System.out.println("Surface area of sphere= "+ sa.area(2));
System.out.println("Surface area of cylinder= "+ cia.area(3,4));
}
}
Output
Surface area of cube = 6.0
Surface area of sphere= 50.24
Surface area of cylinder= 12.0
Example Program of Dynamic Polymorphism with Method Overriding
class Shape
{
void draw()
{
System.out.println("Your favorite shape");
}
void numberOfSides()
{
System.out.println("side = 0");
}
}
class Square extends Shape
{
void draw()
{
System.out.println("SQUARE ");
}
void numberOfSides()
{
System.out.println("side = 4 ");
}
}
class Pentagon extends Shape
{
void draw()
{
System.out.println("PENTAGON ");
}
void numberOfSides()
{
System.out.println("side= 5");
}
}
class Hexagon extends Shape
{
void draw()
{
System.out.println("HEXAGON ");
}
void numberOfSides()
{
System.out.println("side = 6 ");
}
}
public class Overriding{
public static void main(String []args){
Square s = new Square();
s.draw();
s.numberOfSides();
Pentagon p = new Pentagon();
p.draw();
p.numberOfSides();
Hexagon h = new Hexagon();
h.draw();
h.numberOfSides();
}
}
Output
SQUARE;
side = 4;
PENTAGON;
side= 5
HEXAGON
side = 6
Applications
Real-time System Design – These systems have complexities that make it very difficult to code and build from scratch. With the help of object-oriented techniques, it is possible to handle and build these integrated complex frameworks.
Object-oriented databases – Instead of RDBMS, these systems use Object-Oriented Database Management Systems (OODBMS). These databases do not store data such as numbers and strings. Instead, they store everything as objects. They have mainly two features – attributes and methods.
Office Automation Systems – It includes all modes of electronic information sharing, which might be business, personal, formal, informal, that involves the exchange of messages between people and machines.
Simulation and Modelling – Complex modeling systems involve understanding and modeling interactions as clearly as possible. Object-oriented methods provide an alternative and simple method for simulating such processes.
Final Thoughts
We have brought to you the 5 fundamental Java OOPS concepts that are essential to understanding further concepts in the language. These 5 Java OOPS concepts have also been illustrated with suitable examples so that it is easy to understand the concepts.
At TechAffinity, our expert Java developers are proficient in providing end-to-end development services based on client needs and requirements. Our experts have developed an innovative approach that helps to develop robust, highly scalable, and comprehensive solutions. Please feel free to line up your queries and email us at media@techaffinity.com or get in touch by scheduling a meeting.
Also Read: Java vs Python