30 Ways to Write Better Python Code – Part 1
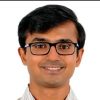
Author : Abishek Surya RS 23rd Jun 2020
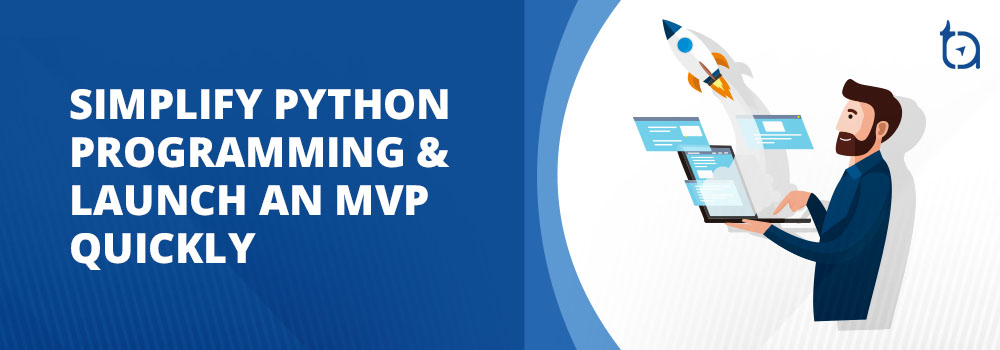
PythonPythonWith dynamic semantics, Python is an object-oriented, interpreted, high-level programming language. Python reduces the maintenance cost of the program as it has simple and easy to learn syntax that emphasizes readability. Thanks to its high-level built-in data structures for making it attractive for Rapid Application Development, as well as for using it as a scripting language. Python supports a wide range of libraries and a few of them are numpy, pandas, matpotlib, scipy, etc. is considered one of the most popular programming languages because of its simplicity and readability. Any new beginner will find it easier to learn Python compared to other languages. If you are such a beginner, the following tips will help you write better Python code.
Once you are familiar with these basic concepts, make them a part of your coding style. Practice them every time you write Python code and make them a part of your coding style. So, let’s start with the coding tricks that will help you write better code in Python and improve your overall coding experience as well.
Note: Please compile your code here for the best results.
1) Multiple Assignment for Variables
You can assign values to multiple variables in a single line. The variables are separated by commas. You can assign multiple values to a single variable or multiple variables can be assigned a single value.
For example, let us assign the values 100 and 200 to variables x and y respectively. Now, this is how a normal assignment works.
Output
(100, 200)
<type ‘int’>
<type ‘int’>
Now, with Python, you can assign the variables in a single line. Therefore, we have different scenarios.
Case 1 – Values Equals Variables
When the number of variables equals the number of values, each value will be stored in the respective variable.
Output
(100, 200)
<type ‘int’>
<type ‘int’>
Case 2 – When the Number of Values is Greater than the Number of Variables
When there are more values than the variables, use the asterisk sign to indicate the variable which will store more than one value.
For example,
Output
100
[200 300]
<class ‘int’>
<class ‘list’>
Here, the first value is assigned to the first variable and the remaining values are assigned to the other variable, making it a list type object.
Case 3 – Same Value to Multiple Variables
You can assign one value to more than one variable in a single line using an equal to sign.
For example,
Output
(150, 150, 150)
<type ‘int’>
<type ‘int’>
<type ‘int’>
2) Swapping the Values of Variables
You can swap the values of two variables quickly using Python. But before that, let’s understand how Python makes this process simple. Swapping is the process by which two variables exchange their values.
Case 1 – Swapping Using a Temporary Variable
Output
(100, 200)
(‘After swapping:’, 200, 100)
Case 2 – Swapping Without a Temporary Variable
The below code swaps variables without using a temporary variable.
Output
(100, 200)
(‘After swapping:’, 200, 100)
Case 3 – Python’s Way of Swapping Variables
Also, you can swap variables using the concept of swapping in a single line of code.
For example,
Output
(100, 200)
(‘After swapping:’, 200, 100)
3) String Reverse
You can reverse a string in Python using a simple command. This concept is popularly known as string slicing. After the variable name, you need to use the [::-1] symbol to reverse the string.
For example,
Output
GNIRTS EUQINU
Suggested Read : What is Python used for in Startups? Why Should a CTO Choose Python for Business in 2020?
4) Splitting Words in a Line
You can use the default available library method to split the words in a line. Python does this for you using the split() function or you can write your own method.
Case 1 – Using split() function
Output
[‘This’, ‘is’, ‘a’, ‘unique’, ‘string’, ‘in’, ‘Python’]
Case 2 – Using Iterations
Output
[‘This ‘, ‘is ‘, ‘a ‘, ‘unique ‘, ‘string ‘, ‘in ‘, ‘Python’]
5) Join the Words Given in a List
As the name suggests, this is the exact opposite of the previous function. Here you join a list of words that are going to be a part of a sentence or a phrase. This is done with the help of the join() method.
Output
This is a unique string in Python
6) Find the Most Frequent Element in a List
The element which occurs the maximum number of times is the most frequent element in a list. Below is an example code snippet for better illustration.
Output
2
As you can see, the element 2 in the list occurs 3 times and hence is chosen as the frequent element.
Also Read: How to Create, Access, Slice, Change, Delete & Search Python Arrays?
7) Occurrences of All Elements in a List
For determining the occurrences of each element in a list, you need to use the Collections module in Python. This module has some of the wonderful features that developers find really useful. Here, the Counter method gives a key, value pair. For our purpose, this serves as a key and its occurrence pair.
Output
Counter({2: 3, 1: 2, 3: 1, 4: 1, 5: 1})
8) Join Strings Using the Addition (+) Operator
You can join several strings at one time using the plus operator or the concatenation operator.
Output
Hire Python Development Teams.
9) More than One Conditional Operator
You can combine two or more conditional operators in a single line of code with the help of the logical operators. However, this can also be done using a method called chaining the operators. For example, if you need to check if the value of a variable lies between 50 and 100, normally the code looks like.
Output
Be Future Ready.
But with operator chaining, this is written as,
Output
Be Future Ready.
Since the condition is satisfied, the operator chaining works effectively and the text is displayed on the screen.
10) Printing a String Multiple Times
You can use the multiplication operator, to print a given string multiple times. This is a useful way to repeat a string several times.
Output
Hire Python Developers from TechAffinity.
Hire Python Developers from TechAffinity.
Hire Python Developers from TechAffinity.
Closing Thoughts
You can use this as a starting point to learn more about the good coding practices that will help you as part of your developer journey. We hope that you have been able to learn or remember some of the basic concepts that you might have missed or not paid enough attention to. We hope to keep you updated in the next parts of the series, which will help you even further. To stay updated with our next post on this series, we encourage you to subscribe to our newsletter.
At TechAffinity, our Python development team has experts who are always updating themselves and delivering the best Python services to our clients. If you have any queries, share them with media@techaffinity.com or schedule a meeting with our experts.
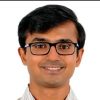