3 Commonly Misunderstood OOPS Concepts in Java
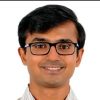
Author : Abishek Surya RS 29th Jun 2023
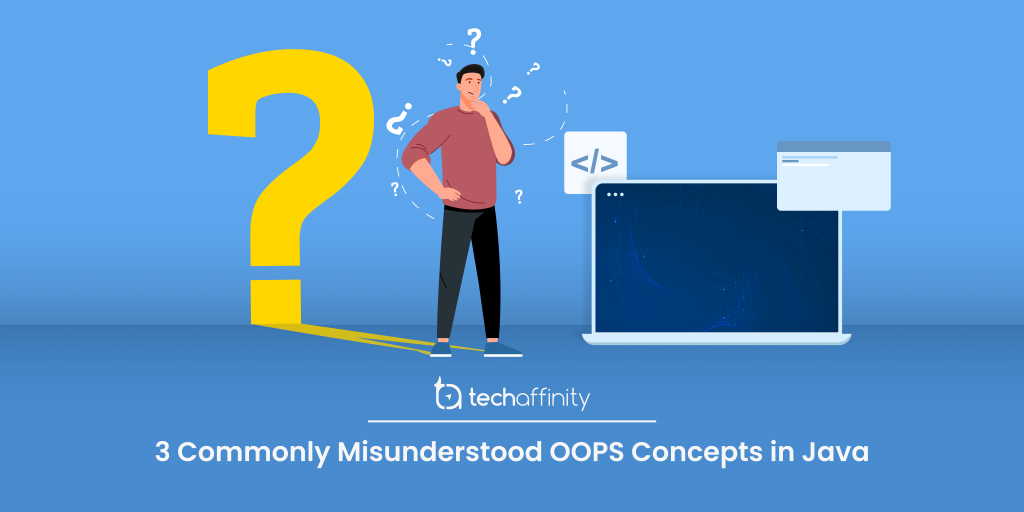
In the dynamic world of software development, leveraging Object-Oriented Programming (OOP) concepts is crucial for businesses seeking efficient, maintainable, and scalable solutions. Java, with its robust OOP capabilities, empowers developers to create code that aligns with their business needs. In this article, we will illustrate 3 commonly misunderstood OOPS concepts in Java – Class(‘this’ keyword usage), inheritance, and polymorphism – and their real-life applications across various industries.
Looking for an introduction to the major OOPS concepts? Then, read this first.
The ‘this’ Keyword: Code Clarity and Precision
The ‘this’ keyword plays a vital role in enhancing code clarity and precision. It ensures correct access to variables within a class to avoid variable ambiguity, reducing errors and improving collaboration among developers.
Let’s take the example of a customer management system:
class Customer {
private String name;
private int age;
public void setName(String name, int age) {
this.name = name;
}
public void displayInfo() {
System.out.println("Customer name: " + this.name);
System.out.println("Customer age: " + this.age);
}
}
public class Main {
public static void main(String[] args) {
Customer customer1 = new Customer();
customer1.setName("John Doe");
customer1.setAge(25);
customer1.displayInfo();
}
}
Output:
Customer name: John Doe
Customer age: 25
In this example, the ‘this’ keyword provides access to the correct instance variables ‘name’ and ‘age’ within the ‘displayInfo()’ method, resulting in the proper display of the customer’s name and age.
By using ‘this’ keyword, we improve code readability, and minimize the potential for errors caused by variable clashes.
Inheritance: Code Reusability and Structure
Inheritance is a fundamental OOPS concept that enables code reusability and promotes structured code organization. It allows us to create new classes by inheriting properties and behaviors from existing classes, reducing redundancy, and accelerating development cycles.
Let’s look at a product management system:
class Product {
protected String name;
protected double price;
public Product(String name, double price) {
this.name = name;
this.price = price;
}
public void displayInfo() {
System.out.println("Name: " + name);
System.out.println("Price: $" + price);
}
}
class ElectronicProduct extends Product {
private String brand;
public ElectronicProduct(String name, double price, String brand) {
super(name, price);
this.brand = brand;
}
@Override
public void displayInfo() {
super.displayInfo();
System.out.println("Brand: " + brand);
}
}
public class Main {
public static void main(String[] args) {
Product product = new Product("Widget", 19.99);
product.displayInfo();
System.out.println("------------------");
ElectronicProduct electronicProduct1 = new ElectronicProduct("Smartphone", 499.99, "ABC Electronics");
electronicProduct.displayInfo();
}
}
Output:
For the Product instance:
Name: Widget
Price: $19.99
For the ElectronicProduct instance:
Name: Smartphone
Price: $499.99
Brand: ABC Electronics
In this example, the Product class serves as the base class, providing common properties and behaviors. The ElectronicProduct class inherits from Product(name, price) and adds its own specific property (brand).
By leveraging inheritance, we achieve code reusability, as the common functionalities of products are encapsulated in the base class, while the specialized features of electronic products are added in the derived class. This structured hierarchy enhances code organization, maintainability, and scalability.
Polymorphism: Flexibility and Adaptability
Polymorphism, another powerful OOPS concept, empowers businesses to build flexible and adaptable software solutions. It helps to write code that can seamlessly work with different types of objects, enabling agility and future-proofing.
Let’s consider the example of payment processing:
interface Payment {
void processPayment();
}
class CreditCardPayment implements Payment {
@Override
public void processPayment() {
System.out.println("Processing credit card payment...");
// Logic for credit card payment processing
}
}
class PayPalPayment implements Payment {
@Override
public void processPayment() {
System.out.println("Processing PayPal payment...");
// Logic for PayPal payment processing
}
}
public class Main {
public static void main(String[] args) {
Payment payment1 = new CreditCardPayment();
payment1.processPayment();
System.out.println("------------------");
Payment payment2 = new PayPalPayment();
payment2.processPayment();
}
}
Output:
Processing credit card payment...
Processing PayPal payment...
In this example, the Payment interface defines a common method processPayment(). The CreditCardPayment and PayPalPayment classes implement this interface with their specific payment processing logic.
By utilizing polymorphism and referencing objects through the interface, you can seamlessly switch between different payment methods, providing flexibility and scalability in your code.
Conclusion:
By leveraging these OOPS concepts in real-life business scenarios, such as e-commerce, banking, and healthcare, businesses can optimize their software development processes, respond swiftly to market demands, and deliver high-quality solutions tailored to their specific needs. Embracing the power of OOPS concepts in Java development empowers businesses to stay competitive, drive innovation, and achieve long-term success in the dynamic digital landscape.Our Java developers can provide you with cutting-edge solutions with their expertise in web application development services and mobile app development services. Unlock new possibilities for your business by sending your queries to media@techaffinity.com and our team will get in team with you promptly.
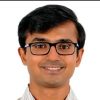