What’s New for Developers in JavaScript ES11 | Approved ECMAScript 2020 Spec for JavaScript
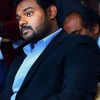
Author : John Prabhu 22nd Jun 2020
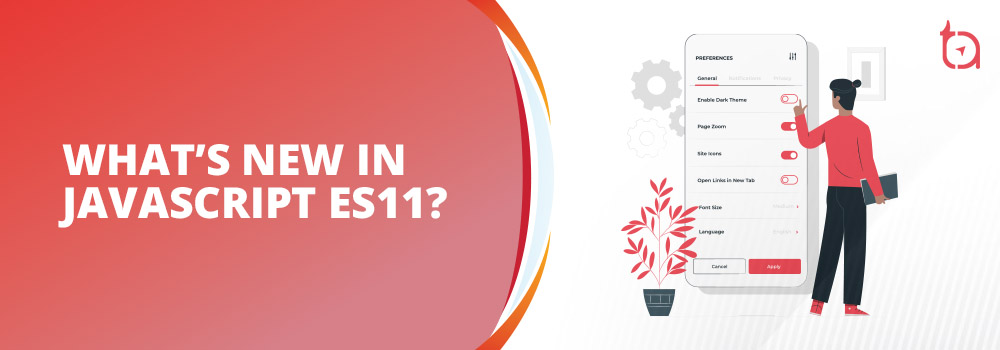
According to Statista’s “Most used programming languages among developers worldwide, as of early 2020” report, 67.7% of developers across the globe use JavaScript and it is the top programming language. In 2020, new features are added to JavaScript ES11 (also known as ECMAScript 2020)
Before the arrival of JavaScriptJavaScriptJavaScript, often abbreviated as JS, is a high-level, interpreted scripting language that conforms to the ECMAScript specification. JavaScript has curly-bracket syntax, dynamic typing, prototype-based object-orientation, and first-class functions. ES6 (ECMAScript 2015), JavaScript language development happened very slowly. After the ES6 update, new advanced features are added each year. It is also important to note that modern browsers might not support all of the JavaScript features. But with the help of transpilers like Babel, you can use them. Let’s take a look at the new features updated to the JavaScript language in 2020.
1. Optional Chaining
As a JavaScript developer, you must have come across this error a thousand times.
TypeError: Cannot read property ‘z’ of undefined
It happens because you tried to access a property on something that is not an object.
Accessing an Object Property
You will get similar errors from the JavaScript engine in such scenarios. However, there are situations wherein it doesn’t require a value because you might know it will be. Luckily, here is another optional chaining feature. You can use the optional chaining operator that can be represented using a question mark immediately followed by a dot (“?.”). It commands the JavaScript engine to not throw an error but return “undefined” as output.
Also, you can use this optional chaining feature while calling a function or accessing array values.
Accessing an Array
Calling a Function
2. Nullish Coalescing
If you want to use a fallback value, you have to use a logical operator (“||”). It applies to almost all cases, but there are exceptions in some scenarios. Suppose the initial value is a number or a Boolean. Below is an example in which we will be assigning a number to a variable, or default it to 5 if the initial value is not a number.
The ‘number’ variable is equal to 7 because the left hand value (theNumber) is truthy (i.e., 7). But what if the ‘number’ value is not 7, and is assigned to 0?
Since 0 is not a positive/natural number, it is a falsy value. As a result, the ‘number’ variable will now get the right-hand value assigned to it, which is 5. Anyway, that is not what we want. Luckily, without writing additional codes and checks to confirm ‘theNumber’ variable is a number, you can use the nullish coalescing operator that can be written using two question marks – ‘??’.
The right-hand side value will only be assigned if the left-hand side value is equal to undefined or null. Hence, in the example above, the ‘number’ variable is equal to 0. Here’s what happens when ‘theNumber’ variable is equal to null:
Suggested Read: JavaScript Still the Most Popular Programming Language
3. Private Fields
Many programming languages have classes that allow defining class properties, such as protected, private, and public. Public properties can be accessed both from outside a class and its subclasses; whereas, protected classes can be accessed only by its subclasses. On the other hand, private properties can only be accessed from inside of a class. Since ES6, JavaScript started supporting class syntax, but private fields are now supported. To define a private property in JavaScript, it should have the hashtag symbol in the prefix.
If you try to access a private property from outside, the JavaScript engine will return an error.
4. Static Fields
To use a class method, a class had to be instantiated first, as shown below.
When you try to access a method without instantiating the ‘Smartphone’ class, it will result in an error. With the help of static fields, you can now declare a class method using static keyword and call it from outside of a class.
5. Top Level Await
To wait for a Promise to complete, a function with await operator should be defined within async function.
On the downside, if there is a need to wait for something in the global scope, it would not be possible, and generally needs an Immediately Invoked Function Expression.
With the help of Top Level Await, you don’t have to wrap code in an async function. Instead, the below code will work:
const color = await fetch(silver)
It is very useful when it comes to using a fallback source when the initial one fails or resolving module dependencies.
Also Read: How to Effectively Write JavaScript Arrow Functions
6. Promise.allSettled
When you want multiple Promises to complete, you can use Promise.all ([promise_1, promise_2]). In this process, if one of the promises fails, the JavaScript engine will throw an error. Luckily, there are cases in which the failure of one promise doesn’t matter, and the rest would resolve. To achieve that, the new JavaScript ES11 unpacks Promise.allSettled.
Hence, only a resolved Promise returns an object with status and value; whereas, rejected Promise returns an object with status and reason.
7. Dynamic Import
While using webpack for module bundling, you may have used dynamic imports. With JavaScript ES11, you get native support for this feature.
Since many applications are using module bundlers such as webpack for optimizing and transpiling code, this feature has come a little late as an update.
8. MatchAll
MatchAll method is useful when it comes to finding all the matches and their positions by applying the regular expression to a string. On the other hand, the match method returns only the items that were matched.
Output
// ‘iPhone’
// ‘iPhone’
// ‘iPhone’
matchAll in contrast, returns additional information, such as the index of the string found.
Output is:
0: “iPhone”
1: “iPhone”
groups: undefined
index: 10
input: “S series, iPhone, note series, iPhone, A series, iPhone, Moto phones”
0: “iPhone”
1: “iPhone”
groups: undefined
index: 31
input: “S series, iPhone, note series, iPhone, A series, iPhone, Moto phones”
0: “iPhone”
1: “iPhone”
groups: undefined
index: 49
input: “S series, iPhone, note series, iPhone, A series, iPhone, Moto phones”
You Might be Interested: Avoid these 5 Typical JavaScript Errors
9. globalThis
You can execute JavaScript codes in different environments, such as browsers or Node.jsNode.jsNode.js is a cross-platform runtime environment used to develop server-side and networking applications. It is an open source platform and the applications are developed using JavaScript. It can run within the Node.js runtime on Microsoft Windows, Linux, and OS X.. In browsers, a global object is available under the window variable; whereas, in Node.js, it is an object called global. With globalThis, it is now easy to use a global object regardless of the environment in which the code is running.
10. BigInt
2^53 – 1 is the maximum number you can represent in JavaScript. But with JavaScript ES11 update, BigInt will support the creation of numbers that are bigger than that. You can view the maximum number that can be represented in JavaScript for yourself by executing the below code.
Please Note: The right output is 9007199254741001.
Hence, when you implement BigInt by adding the alphabet ‘n’ at the end of your number, you will get the correct output/result.
Also, it is important to not mix BigInt with other types:
Suggested Read: 5 Popular JavaScript Frameworks
Wrapping Up:
As said earlier, while discussing “Dynamic Import”, you might be using a separate tool such as webpack, which can potentially slow down your website/web app. Don’t let your website/web app slow down, and here’s why. Take advantage of the new native features announced and available for use in JavaScript ES11 and boost your web project’s efficiency. Also, we wish you a happy “JavaScripting” with your web project.
We, at TechAffinity, handle complex websites and web apps with proficient JavaScript developers who have hands-on experience in implementing new JavaScript features to web projects. If you want to build a new web project or improvise your web projects concerning JavaScript development, you can shoot an email to media@techaffinity.com or schedule a call with our expert team.
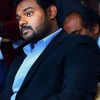